Note
Go to the end to download the full example code.
Geodesic reconstruction#
This example shows how to use the geodesic reconstruction
import matplotlib.pyplot as plt
import matplotlib as mpl
import numpy
import spam.excursions
import spam.filters
Generate a morphology#
Generation and excrusion of a realisation of a correlated random field
covarianceParameters = {'len_scale': 0.005}
realisation = spam.excursions.simulateRandomField(nNodes=500, covarianceParameters=covarianceParameters, nRea=1, dim=2, vtkFile="morpho")
excursion = realisation[0] > 0.05
Generating morpho_0000... 7.76 seconds
Geodesic reconstruction#
Direct definition of the marker (square in the center)
marker = numpy.zeros(excursion.shape, dtype=bool)
marker[230:270, 230:270] = excursion[230:270, 230:270]
rec_center = spam.filters.binaryGeodesicReconstruction(excursion, marker)
Definition of the marker with a list of plans
marker = [0, 0] # direction 0 distance 0 (top side)
rec_top = spam.filters.binaryGeodesicReconstruction(excursion, marker)
marker = [1, 0, 0, -1] # direction 1 distance 0 (left side) and direction 0 distance max (bottom side)
rec_botleft = spam.filters.binaryGeodesicReconstruction(excursion, marker)
Plots
plot_image = numpy.zeros(excursion.shape, dtype=int)
plot_image[numpy.where(excursion)] = -1 # light blue for the morphology
plot_image[numpy.where(rec_center)] = -2 # dark blue for the center marker
plot_image[numpy.where(rec_top)] = 1 # orange for the top side marker
plot_image[numpy.where(rec_botleft)] = 2 # red for the bottom/left side marker
plt.figure()
plt.imshow(plot_image, cmap='coolwarm')
plt.plot([-5, -5], [0, 499], lw=3, ls='--', color='red')
plt.plot([0, 499], [504, 504], lw=3, ls='--', color='red')
plt.plot([0, 499], [-5, -5], lw=3, ls='--', color='orange')
plt.plot([230, 230, 270, 270, 230], [230, 270, 270, 230, 230], lw=3, ls='--', color='blue')
plt.title("Morphology and Geodesic reconstruction for different markers")
plt.axis('off')
plt.show()
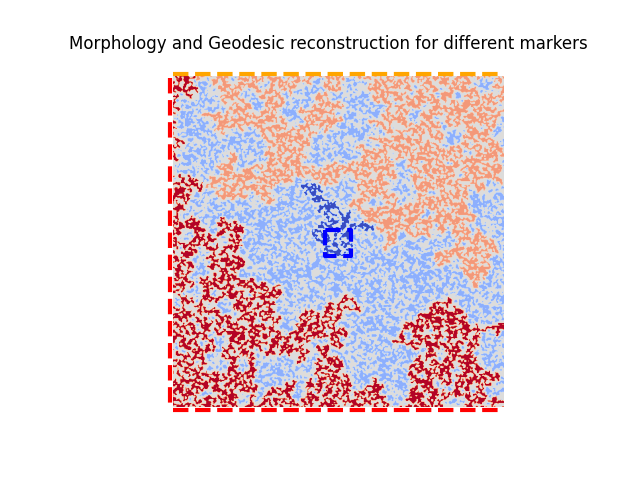
Notes#
The geodesic reconstruction is implemented for 2D and 3D binary morphologies
Total running time of the script: (0 minutes 8.210 seconds)