Note
Go to the end to download the full example code
Project a scalar field onto an unstructured mesh#
This example shows how to generate a correlated random field project it onto an unstructured mesh
import matplotlib.pyplot as plt
import spam.excursions
import spam.mesh
Create the random field#
Generate a realisation of the correlated (Gaussian centered) Random Field in a cube of size 1x1x1
covarianceParameters = {
"var": 1.0,
"len_scale": 0.5,
}
nNodes = 100 # discretisation of the field
realisation = spam.excursions.simulateRandomField(nNodes=nNodes, covarianceParameters=covarianceParameters, dim=3)
plt.figure()
plt.imshow(realisation[0][nNodes // 2, :, :])
plt.title("2D Slice of the field")
plt.show()
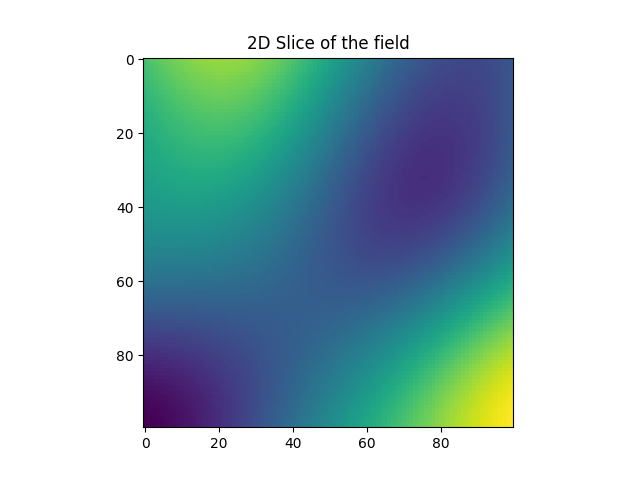
Generate a cylindrical mesh#
# Set the geometrical properties of the mesh
centre = [0.5, 0.5]
radius = 0.5
height = 1.0
lcar = 0.1 # average distance between nodes
points, connectivity = spam.mesh.createCylinder(centre, radius, height, lcar, vtkFile="cylinder")
# points, connectivity = spam.mesh.createCuboid([1, 1, 1], lcar, vtkFile="cube")
Projection#
Set the geometrical properties of the mesh
field = {
"origin": [0, 0, 0],
"lengths": [1, 1, 1],
"nCells": [nNodes - 1, nNodes - 1, nNodes - 1],
"values": realisation,
}
thresholds = [0.2]
materials = spam.mesh.projectField(
{"points": points, "cells": connectivity},
field,
thresholds=thresholds,
writeConnectivity="spam_projection",
vtkMesh="Hello",
)
Shift connectivity by 1 to start at 1
Returns the connectivity matrix and the material repartition
print(f"Connectivity matrix: {connectivity.shape} -> (node 1, node 2, node 3, node 4)")
print(f"Materials: {materials.shape} -> (phase number, sub volume, orientation x, orientation y, orientation z)")
Connectivity matrix: (4044, 4) -> (node 1, node 2, node 3, node 4)
Materials: (4044, 5) -> (phase number, sub volume, orientation x, orientation y, orientation z)
Notes#
One can generate several fields and set them in the list
field["values"]
line 46. The same number of thresholds have to be set on line 48.
Total running time of the script: (0 minutes 28.319 seconds)